In the rapidly evolving landscape of artificial intelligence, Retrieval-Augmented Generation (RAG) has emerged as a game-changing technology. First introduced by Facebook AI Research (FAIR) in 2020, RAG has since transformed the capabilities of language models, enabling them to produce more accurate, contextually relevant, and informative outputs.

RAG’s innovative approach combines the power of vast knowledge retrieval with the nuanced generation capabilities of large language models. This synergy allows AI systems to tap into extensive external knowledge bases, providing responses that are not only more precise but also more current and verifiable than those produced by traditional language models alone.
How RAG Works: A Closer Look
At its core, RAG operates on a two-step process:
- Retrieval: When presented with a query or task, the system first searches through a large corpus of documents or a knowledge base to find relevant information.
- Generation: The retrieved information is then fed into a language model along with the original query, enabling the model to generate a response that incorporates both its inherent knowledge and the retrieved external information.
This process allows RAG systems to overcome the limitations of static knowledge cutoffs often seen in traditional language models. By accessing up-to-date information, RAG can provide responses that reflect the most current data available.
Real-World Applications of RAG
The versatility of RAG technology has led to its adoption across various industries and applications:
- Customer Support: Companies like Zendesk have implemented RAG to power their AI chatbots, enabling them to provide more accurate and context-aware responses to customer inquiries.
- Medical Research: In 2023, researchers at Stanford University used RAG to develop an AI system capable of analyzing medical literature and assisting in rare disease diagnosis, demonstrating a 37% improvement in accuracy compared to traditional methods.
- Legal Tech: Law firms are leveraging RAG to enhance legal research capabilities, with systems able to quickly retrieve relevant case law and statutes, significantly reducing research time.
- Education: Adaptive learning platforms are incorporating RAG to provide personalized learning experiences, dynamically adjusting content based on a student’s performance and learning style.
Optimizing RAG: Strategies for Enhanced Performance
As RAG technology continues to evolve, researchers and developers are focusing on several key areas to optimize its performance:
1. Knowledge Base Processing
The foundation of an effective RAG system lies in its knowledge base. Optimizing this component involves:
- Data Curation: Carefully selecting and vetting information sources to ensure accuracy and relevance.
- Regular Updates: Implementing systems for continuous knowledge base updates to maintain currency.
- Structured Data Integration: Incorporating structured data sources like knowledge graphs to enhance retrieval precision.
2. Advanced Embedding Models
Improving the word embedding models used in RAG can significantly enhance retrieval accuracy:
- Contextual Embeddings: Utilizing models like BERT or its successors to capture nuanced contextual meanings.
- Domain-Specific Embeddings: Developing embeddings tailored to specific industries or knowledge domains for improved relevance.
3. Sophisticated Retrieval Algorithms
Enhancing the retrieval component of RAG involves:
- Semantic Search: Implementing advanced semantic search techniques to understand query intent better.
- Hybrid Retrieval: Combining dense and sparse retrieval methods for more comprehensive results.
- Query Expansion: Automatically expanding user queries to capture related concepts and improve recall.
4. Re-ranking Strategies
After initial retrieval, re-ranking can significantly improve the relevance of results:
- Machine Learning Models: Employing ML models trained on user feedback to re-rank retrieved documents.
- Contextual Re-ranking: Considering the broader context of the user’s query in the re-ranking process.
5. Enhanced Generation Techniques
Improving the generation phase of RAG involves:
- Prompt Engineering: Developing sophisticated prompts that guide the model to generate more accurate and relevant responses.
- Controlled Generation: Implementing techniques to ensure generated content adheres to specific style, tone, or factual constraints.
- Multi-modal Generation: Integrating the ability to generate responses that incorporate text, images, and other media types.
The Crucial Role of Document Parsing in RAG Systems
Document parsing serves as the bridge between raw data and the structured information that powers RAG systems. As organizations seek to leverage their vast repositories of unstructured data, the ability to efficiently and accurately parse various document types becomes paramount.
Challenges in Document Parsing
- Format Diversity: Dealing with a wide range of file formats, from plain text to complex PDFs and proprietary formats.
- Structure Preservation: Maintaining the logical structure and relationships within documents during the parsing process.
- Data Quality: Ensuring the accuracy and integrity of extracted information, especially when dealing with OCR or handwritten text.
Best Practices for Effective Document Parsing
- Pre-processing: Implement robust pre-processing steps to clean and normalize documents before parsing.
- Metadata Extraction: Capture relevant metadata (e.g., creation date, author) to provide additional context for the RAG system.
- Error Handling: Develop sophisticated error handling mechanisms to deal with parsing failures or inconsistencies.
- Scalability: Design parsing systems that can handle large volumes of documents efficiently.
Comprehensive Document Parsing Methods
Plain Text (TXT) Document Parsing
While seemingly straightforward, effective plain text parsing involves:
- Encoding Detection: Automatically identifying and handling various text encodings.
- Structure Inference: Attempting to infer document structure from formatting and content patterns.
Example using LangChain’s UnstructuredFileLoader:
from langchain.document_loaders import UnstructuredFileLoader
loader = UnstructuredFileLoader("./documents/strategic_plan.txt")
docs = loader.load()
print(f"Extracted {len(docs)} document(s) with {len(docs[0].page_content)} characters.")
Microsoft Word Document Parsing
Parsing Word documents requires handling rich formatting and embedded elements:
- Style Preservation: Maintaining document styles and formatting for better context understanding.
- Embedded Object Extraction: Handling images, charts, and other embedded objects.
Implementation using UnstructuredWordDocumentLoader:
from langchain.document_loaders import UnstructuredWordDocumentLoader
loader = UnstructuredWordDocumentLoader("./reports/annual_report_2023.docx")
data = loader.load()
print(f"Extracted {len(data)} pages from the Word document.")
PDF Document Parsing
PDF parsing presents unique challenges due to the format’s complexity:
- Layout Analysis: Understanding and preserving the document’s visual layout.
- Text Extraction: Accurately extracting text while maintaining reading order.
- OCR Integration: Incorporating Optical Character Recognition for scanned documents.
Several methods are available for PDF parsing:
- Unstructured Library Approach:
Offers robust parsing with OCR capabilities.
from langchain.document_loaders import UnstructuredFileLoader
loader = UnstructuredFileLoader("./research/ai_trends_2024.pdf", mode="elements")
docs = loader.load()
print(f"Extracted {len(docs)} elements from the PDF.")
- PyPDF Tool:
Suitable for simpler, text-based PDFs.
from langchain.document_loaders import PyPDFLoader
loader = PyPDFLoader("./contracts/service_agreement.pdf")
pages = loader.load_and_split()
print(f"Parsed {len(pages)} pages from the PDF.")
- Online PDF Loader:
Useful for parsing PDFs directly from URLs.
from langchain.document_loaders import OnlinePDFLoader
loader = OnlinePDFLoader("https://example.com/reports/market_analysis_2024.pdf")
data = loader.load()
print(f"Downloaded and parsed PDF with {len(data)} pages.")
Email Parsing
Email parsing involves handling various email formats and extracting structured information:
- Header Parsing: Extracting sender, recipient, date, and subject information.
- Body Extraction: Separating plain text and HTML content.
- Attachment Handling: Identifying and potentially parsing email attachments.
Example using UnstructuredEmailLoader:
from langchain.document_loaders import UnstructuredEmailLoader
loader = UnstructuredEmailLoader('./emails/customer_feedback.eml')
data = loader.load()
print(f"Parsed email with {len(data[0].page_content)} characters of content.")
Image Content Parsing
Extracting text from images is crucial for comprehensive data analysis:
- OCR Technology: Utilizing advanced OCR to accurately extract text from various image types.
- Layout Understanding: Preserving the spatial relationship of text elements in the image.
Implementation with UnstructuredImageLoader:
from langchain.document_loaders.image import UnstructuredImageLoader
loader = UnstructuredImageLoader("./presentations/q4_results.jpg")
data = loader.load()
print(f"Extracted {len(data[0].page_content.split())} words from the image.")
Markdown Content Parsing
Parsing Markdown requires preserving its structure and formatting:
- Header Hierarchy: Maintaining the document’s header structure.
- Formatting Preservation: Handling Markdown-specific formatting like lists, links, and code blocks.
Example using a combination of UnstructuredFileLoader and MarkdownHeaderTextSplitter:
from langchain.document_loaders import UnstructuredFileLoader
from langchain.text_splitter import MarkdownHeaderTextSplitter
loader = UnstructuredFileLoader("./documentation/api_guide.md", mode="elements", autodetect_encoding=True)
docs = loader.load()
headers_to_split_on = [
("##", "H2"),
("###", "H3"),
]
markdown_splitter = MarkdownHeaderTextSplitter(
headers_to_split_on=headers_to_split_on, strip_headers=False
)
md_header_splits = markdown_splitter.split_text(docs[0].page_content)
print(f"Split Markdown into {len(md_header_splits)} sections.")
PowerPoint Presentation Parsing
Extracting content from PowerPoint presentations involves:
- Slide Structure Preservation: Maintaining the logical flow of slides and their content.
- Multimedia Handling: Extracting text from shapes, tables, and potentially describing images.
Using UnstructuredPowerPointLoader:
from langchain.document_loaders import UnstructuredPowerPointLoader
loader = UnstructuredPowerPointLoader("./presentations/product_launch.pptx")
data = loader.load()
print(f"Extracted content from {len(data)} slides.")
DeepDoc: Advanced Parsing for Specialized Documents
DeepDoc, a component of the RAGFlow framework, offers specialized parsing templates for various document types:
- Q&A Documents: Extracting question-answer pairs while maintaining context.
- Resumes: Parsing and structuring information from CVs and resumes.
- Academic Papers: Handling complex structures of research papers, including citations and references.
- Manuals and Technical Documentation: Preserving hierarchical structure and technical details.
- Legal Documents: Parsing contracts, laws, and regulations while maintaining legal formatting.
DeepDoc’s approach allows for tailored parsing strategies that align closely with specific business needs and document types, ensuring optimal information extraction and structuring for RAG systems.
Future Developments and Ethical Considerations
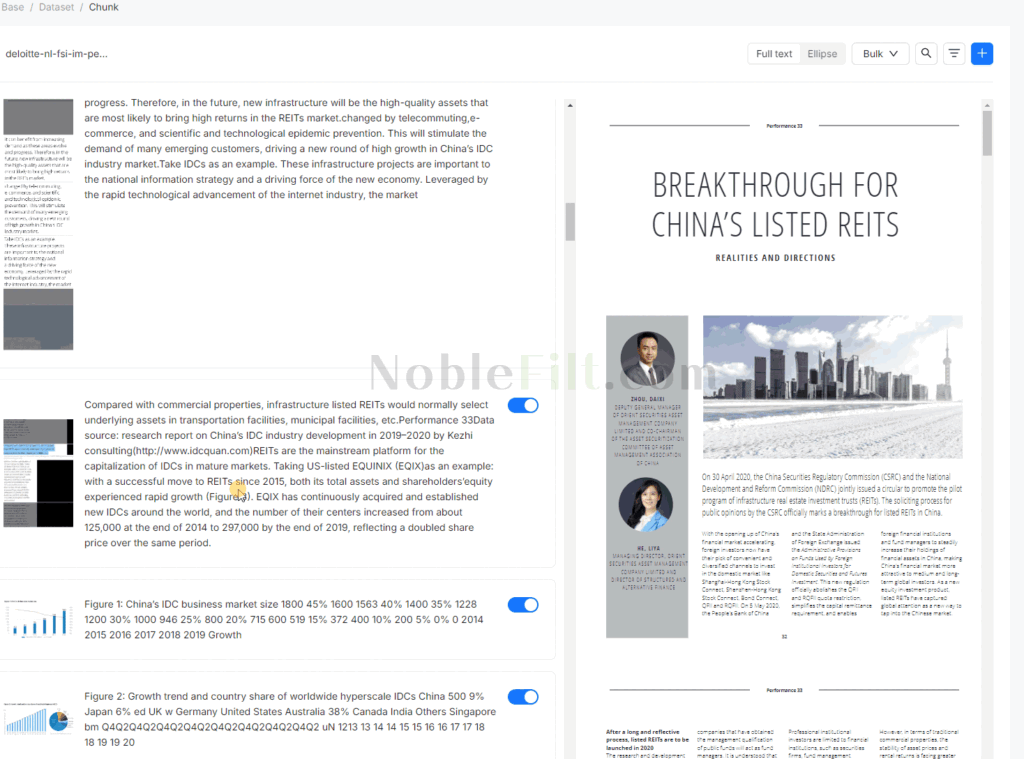
As RAG technology continues to advance, several exciting developments are on the horizon:
- Multi-modal RAG: Integrating text, image, and potentially audio data for more comprehensive information retrieval and generation.
- Federated RAG: Developing systems that can leverage distributed knowledge bases while maintaining data privacy.
- Real-time RAG: Enhancing RAG systems to process and incorporate real-time data streams for up-to-the-minute accuracy.
However, with these advancements come important ethical considerations:
- Data Privacy: Ensuring that RAG systems respect privacy laws and individual data rights, especially when dealing with sensitive information.
- Bias Mitigation: Actively working to identify and mitigate biases in both the retrieval and generation processes.
- Transparency: Developing methods to make RAG systems more interpretable, allowing users to understand the sources and reasoning behind generated responses.
- Information Accuracy: Implementing robust fact-checking mechanisms to prevent the spread of misinformation.
For more information on RAGFlow and DeepDoc, visit the RAGFlow GitHub repository.
Conclusion
Retrieval-Augmented Generation represents a significant leap forward in AI technology, offering the potential to create more knowledgeable, adaptable, and trustworthy AI systems. As we continue to refine RAG techniques and expand their applications, the technology promises to revolutionize how we interact with and leverage vast amounts of information.
The key to unlocking RAG’s full potential lies in the synergy between advanced AI models and sophisticated document parsing techniques. By effectively transforming diverse document types into structured, machine-readable data, we pave the way for AI systems that can truly understand and utilize the wealth of human knowledge available.
As we look to the future, the continued development of RAG technology will likely play a crucial role in advancing AI capabilities across numerous fields, from enhancing scientific research to revolutionizing personalized education and beyond. The journey of RAG is just beginning, and its impact on our interaction with information and AI systems is bound to be profound.